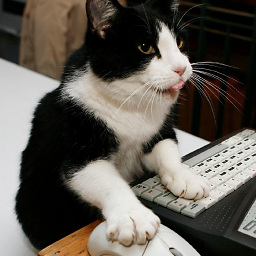
21
Last seen 3 hours ago
Member for 9 months, 12 days
Difficulty Easy
Medium Python programmer. I like clear code and one-liners.
Strings and Integers

Oneliner
Count Vowels
1
15
0

reversed() and replace()
Reverse Integer
1
5
0

Oneliner
Integer Sign Determination
1
4
2

Oneliner
Leap Year Checking
1
3
0

itertools.permutations
All Permutations
1
0
1

Oneliner
Perfect Number Checking
1
0
0

Twoliner
Quadratic Equation Roots
1
0
0

Oneliner
Count Divisibles in Range (simplified)
1
0
0

Can you solve this in other way?!
Find Remainder
2
6
0

The Great Oneliner
Longest Common Prefix
2
0
1

Use regex
Count Substring Occurrences
2
0
0

num in list?
Armstrong Number Checking
2
0
0

Oneliner
The Longest Word
3
0
0

Twoliner (try-except)
Convert Date
3
0
0

Oneliner
Fuzzy String Matching
3
0
0

First
Excel Column Number
6
0
0

Oneliner
Excel Column Number
7
0
0

Clearest possible solution
Maximum Among Three
8
2
1

Range
Sum of Integers
9
4
0

First
Goes Right After (simplified)
10
0
0

NothingSpecial
Rectangle Perimeter
11
0
0

Use "Math" module!
Number With Exclamation
12
2
0

Use replace()
Replace All Occurrences
12
1
1

First
Just Fizz!
12
0
0

5th — yield
Multiply (Intro)
13
2
0

TheVerySmallSolution
Convert To Title Case
18
1
0

First
Is Even
23
12
2

What is this?!
Multiply (Intro)
25
1
0

lambda
Multiply (Intro)
33
3
0

Oneliner
All Upper I
35
1
0

Oneliner
Cut Sentence
35
0
0

First
First Word (simplified)
53
8
0

AbSuRd
Multiply (Intro)
55
1
1

First
Fizz Buzz
82
2
1

First
Multiply (Intro)
98
0
2

4th f''
Multiply (Intro)
99
0
0

Oneliner
Beginning Zeros
114
0
0

Oneliner
Max Digit
154
0
0

reduce+mul
Digits Multiplication
537
0
0

__import__ + map
Digits Multiplication
538
0
0

First
Backward String
542
0
0

Oneliner
The Most Wanted Letter
567
0
0

len-str
Number Length
645
0
0

Classic
Bird Language
1501
0
0
List but not the least

ReadHints!
Stock Profit
56
0
0

Oneliner
Easy Unpack
64
0
0

Oneliner
Frequency Sorting
75
0
0

Oneliner
The Most Frequent
140
0
0

First
Missing Number
157
0
0

Classic
Backward Each Word
158
0
0

Oneliner
Remove All After
162
3
0

Unpractical but beautiful
Chunk
285
0
0

VerySimple
Compress List
430
0
0

First
Words Order
607
0
0

First
Replace First
650
1
1

First
Absolute Sorting
1020
2
1
Look up in the Dict()

First
Ordinary Cardinals
16
0
0

Oneliner
Move Zeros
18
0
0

One String Solution
YAML. Simple Dict
35
0
0

Recursion
How Deep
77
0
0

Simple
Switch Keys to Values
91
0
0

First
Count Digits
92
0
2

Classic
Golden Pyramid
100
4
0

Oneliner
Best Stock
140
0
0

Oneliner
Popular Words
171
0
0

Oneliner with operator.itemgetter()
Bigger Price
200
0
1

"set" and "Counter" are equal...
Verify Anagrams
203
0
0

match-case
Boolean Algebra
228
0
0

Second
YAML. Simple Dict
292
0
0

First
The Flat Dictionary
292
0
0
Strings Theory

A bunch of one-liners
Revorse the vewels
1
0
1

join and enumerate
Matrix-2-String
3
0
0

Mess
String-2-Matrix
3
0
0

Automatic replace
Adjacent Letters
6
0
0

One String Solution
Is String a Number? (Part II)
7
0
0

Oneliner
Long Pressed
9
0
0

Twoliner
One Switch Strings
9
0
0

Classic
Text Formatting
10
9
1

Oneliner
All Upper II
12
1
0

Brute force...
Champernowne Word
13
0
0

Oneliner
Long Repeat
23
4
1

First
Calculator-I
28
0
0

First
Second Index
103
7
1

Classic
Sun Angle
154
5
1

First
The Nearest Square Number
215
0
1

Oneliner
Common Words
246
0
0

Oneliner
House Password
512
0
0

match + pop & append
Letter Queue
802
0
0
List, list and list again

First
Beat The Previous
5
5
0

Oneliner
Not in Order
6
0
0

Absolutely Unclear
Combining Celebrity Names
20
0
2

Classic
Median of Three
26
0
0

Oneliner
Sum by Type
40
0
0

First
Replace with Biggest
68
0
0

SomethingStrange
Integer Palindrome
80
0
0

dict + for
When "k" is Enough!
85
0
0

Slices
Split List
90
0
0

dict-comp and filter
Convert and Aggregate
110
0
0

Three Lines
Cipher Map
145
0
0

First
Replace Last
182
3
0

First
Split Pairs
188
4
0

First
Determine the Order
189
0
0

Oneliner
Flatten a List
260
0
0

Oneliner
Sort Array by Element Frequency
275
0
0
Different kind of sets

Twoliner
Shorter Set
4
0
0

Simple
Expand Intervals
41
5
0

Classic
Nearest Value
125
5
0

Oneliner
The End of Other
165
0
0
Mine

Incorrect Solution.
Checking Perfect Power
3
0
1

Code-golf!
Funny Addition
29
5
1
Ice Base

One-liner
Count And Say
1
0
0

Oneliner
Count Divisibles in Range
3
0
0

Twoliner
Weak Point
87
0
0

Random is the best!
The Best Number Ever
98
3
1

Oneliner
Caesar Cipher (encryptor)
129
0
0

math.gcd()
The Greatest Common Divisor
177
1
0

First
Disposable Teleports
238
0
0

First
Similar Triangles
393
0
0

First
The Hidden Word
510
0
0

First
Digit Stack
647
0
0

First
The Most Numbers
4313
0
0
GitHub

Oneliner
Secret Message
26
4
0

Oneliner
Monkey Typing
194
0
0

enum.Enum
I Love Python!
226
0
0

sum + zip
The Hamming Distance
1326
0
0

eval + s u m
Restricted Sum
1620
0
0

First
Binary Count
2361
0
0

try-except
Number Base
3017
0
0

First
Roman Numerals
3344
0
0
Everything is Object

Oneliners
Every Person is Unique
11
0
0

Simplest
Voice TV Control
39
3
0

Simplest
3 Chefs
42
4
2

Simplest
Army Units
165
0
0

First
The Healers
167
0
0

First
The Lancers
174
0
0

First
The Vampires
335
0
0

Shortly
The Defenders
360
0
0

Second
The Warriors
460
1
0

First
Army Battles
480
0
0

First
The Warriors
859
0
0
Master of the Time Stone

Code-golf
Days Between
12
3
1

Oneliner
Time Converter (24h to 12h)
44
1
0
Blizzard

Oneliner
Worth of Words
40
0
0

Twoliner
Word Pattern
62
0
0

First
Robot Sort
187
0
0
Rock

Oneliner
Sum Consecutives
15
0
0

Oneliner
Caesar Cipher (decryptor)
25
0
0

First
Sort by Removing
69
1
0

First
Rectangles Union
110
0
0
Escher

Oneliner
The Ship Teams
30
0
0
Not On Map

Something_strange
Length of the String
10
0
0

Simple five-liner
Most Wanted Letter
131
0
0